Function in JavaScript
Read This on Github
⭐ Real World Example
Explain the concept of Function
Imagine you are making a cake. To make a cake, you need to follow a recipe that tells you what ingredients to use and in what order to add them. The recipe is like a function in JavaScript. It takes in certain ingredients (called arguments
), and it produces a cake (called the return value
) as the output.
Now, let's say you want to make another cake, but this time you want to use a different kind of flour and sugar. You can use the same recipe, but just change the arguments (the flour and sugar) to make a different cake. This is similar to how we can use a function with different arguments in JavaScript to get different outputs.
Functions are also useful because they allow us to reuse code. Let's say you have a recipe for chocolate frosting that you want to use on both the chocolate cake and the vanilla cake. You can create a function for the frosting recipe and use it for both cakes, instead of writing out the instructions for the frosting separately for each cake.
⭐ Why we need Function in JavaScript ?
Explain the need of Function in JavaScript
We are calculating the average of different pairs of numbers and adding 1 to the result. We are repeating this process three times, but the only thing that changes between the three calculations is the pair of numbers we are using.
let a = 1;
let b = 2;
let c = 3;
console.log('One plus Average of a and b is', 1 + (a + b) / 2);
console.log('done');
console.log('One plus Average of b and c is', 1 + (b + c) / 2);
console.log('done');
console.log('One plus Average of a and c is', 1 + (a + c) / 2);
console.log('done');
It's a lot of work to repeat the same code three times. We can use a function to make our code more concise and easier to read. We can define a function that takes in two numbers and returns the average of those two numbers. Then, we can call the function three times with different arguments to get the same result as above.
function calculate(x, y) {
console.log('One plus Average of ' +
x + ' and ' + y + ' is ' + (1 + (x + y) / 2));
console.log('done');
}
let a = 1;
let b = 2;
let c = 3;
calculate(a, b);
calculate(b, c);
calculate(a, c);
💥 Explain The Code
-
First, we define a function called calculate that takes in two arguments,
x
andy
. The function has two statements inside it:- The first statement logs a message to the console that includes the values of x and y, as well as the result of calculating 1 + (x + y) / 2.
- The second statement logs the string 'done' to the console.
-
Next, we define three variables:
a
,b
, andc
, and assign them the values 1, 2, and 3 respectively. -
Finally, we call the
calculate
function three times, each time passing it different values forx
andy
.- When we call calculate(a, b), the function uses the values of a and b (which are 1 and 2) as the values for x and y, and it logs the following message to the console:
One plus Average of 1 and 2 is 1.5 done
- When we call calculate(b, c), the function uses the values of b and c (which are 2 and 3) as the values for x and y, and it logs the following message to the console:
One plus Average of 2 and 3 is 2.5 done
- When we call calculate(a, c), the function uses the values of a and c (which are 1 and 3) as the values for x and y, and it logs the following message to the console:
One plus Average of 1 and 3 is 2 done
⚡ Playground
⭐ Syntax Breakdown
Breakdown of Function Syntax
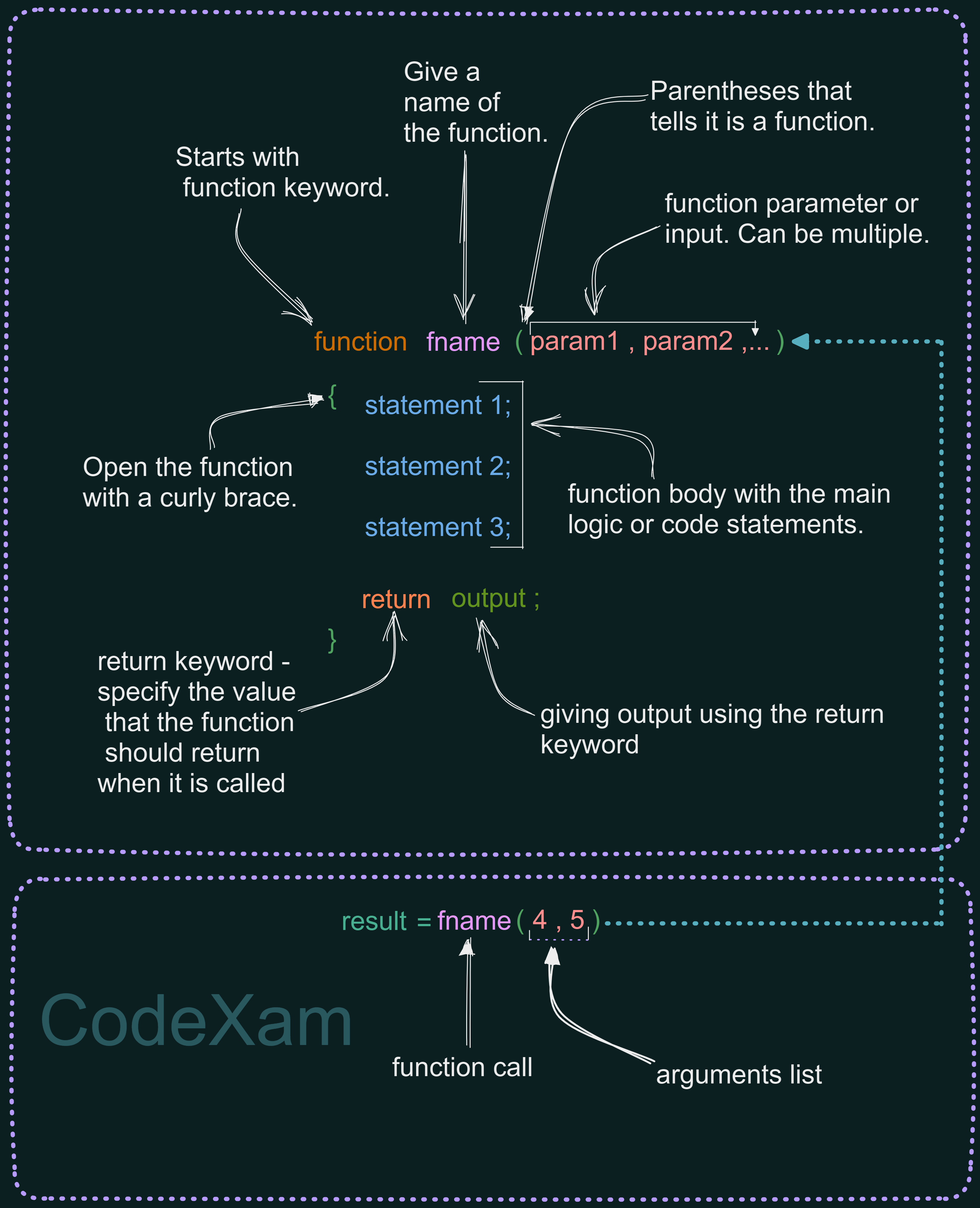
Parameter
-The variables listed in the function's parameters are called the "parameters" of the function.
When you call a function and pass it some values, these values are assigned to the parameters of the function, and the function can use them in its code.
Formal Parameter
- A "formal parameter" is a variable in the function definition that represents the value of an actual parameter passed to the function. It is also called a "parameter"
Argument
- When you call a function and pass it some values, these values are called the "arguments" of the function.
Arguments are the values that you pass to the function when you call it. They are matched up with the function's parameters, and the values of the arguments are assigned to the parameters.
Actual Parameter
- An "actual parameter" is an expression that you pass to a function when you call it. It is also called an "argument"
- It's not necessary to pass arguments to a function. If you don't pass any arguments, the function's parameters will be assigned the value
undefined
. - It's also not necessary to set parameters for a function. If you don't set any parameters, the function will still work, and you can pass arguments to it.
① A function with no parameters and no arguments:
function sayHello() {
console.log('Hello!');
}
sayHello(); // Hello!
② A function with one parameter and one argument:
function sayHello(name) {
console.log('Hello, ' + name + '!');
}
sayHello('Subham'); // Hello, Subham!
③ A function with no parameters and arguments:
function greet() {
console.log("Hello, world!");
}
greet("John"); // prints "Hello, world!"
Math.round()
is a built-in JavaScript function that rounds a number to the nearest integer. It takes one argument, the number to be rounded, and returns the rounded number.
⭐ Modern JavaScript Function
Modern JavaScript Function Syntax
In modern JavaScript, there are several ways to define functions. Here are a few examples:
- Function Declaration
function greet(name) {
console.log("Hello, " + name + "!");
}
This is the traditional way to define a function in JavaScript. The function is declared with the function
keyword, followed by the function name, a list of parameters in parentheses, and the function body in curly braces.
- Function Expression
const greet = function(name) {
console.log("Hello, " + name + "!");
}
In this syntax, the function is assigned to a variable using the const keyword. This is called a "function expression".
- Arrow Function:
const greet = (name) => {
console.log("Hello, " + name + "!");
}
An arrow function is a shorthand syntax for defining a function expression. It is written using the => syntax.
- Arrow function with a single parameter and a concise body
const greet = name => console.log("Hello, " + name + "!");
If the function has a single parameter, you can omit the parentheses around the parameter list. If the function has a single statement in its body, you can omit the curly braces and the return keyword.
- Arrow function with no parameters and a concise body
const greet = () => console.log("Hello, world!");
If the function has no parameters, you must include empty parentheses in the parameter list.
- Arrow function with a single parameter and a block body
const greet = name => { console.log("Hello, " + name + "!"); }
If the function has a single parameter, you can omit the parentheses around the parameter list. If the function has a single statement in its body, you can omit the curly braces and the return keyword.
⚡ Playground
Here are a few key points to note about the differences between these two syntax's:
- The function declaration uses the
function
keyword, while the arrow function uses theconst
keyword to assign the function to a variable. - The arrow function uses the
=>
syntax to define the function, while the function declaration uses the traditionalfunction
keyword. - The function declaration has a
function name
, while the arrow function uses avariable name
. - The function declaration has a set of parentheses around the parameter list, while the arrow function uses parentheses only if the function has one or more parameters.
- The function declaration uses
curly braces
to enclose the function body, while the arrow function uses curly braces only if the function has a block body.
⭐ Example of Function
Example for Beginners
✨ 1. Calculating the sum of two numbers:
const sum = (a, b) => a + b;
console.log(sum(1, 2)); // prints 3
function sum(a, b) {
return a + b;
}
console.log(sum(1, 2)); // prints 3
✨ 2. Concatenating two strings:
const concat = (a, b) => a + b;
console.log(concat("Hello, ", "world!")); // prints "Hello, world!"
function concat(a, b) {
return a + b;
}
console.log(concat("Hello, ", "world!")); // prints "Hello, world!"
✨ 3. Checking if a number is even or odd:
const isEven = n => n % 2 === 0;
console.log(isEven(4)); // prints true
console.log(isEven(5)); // prints false
function isEven(n) {
return n % 2 === 0;
}
console.log(isEven(4)); // prints true
console.log(isEven(5)); // prints false
✨ 4. Retrieving the length of an array:
const getLength = arr => arr.length;
console.log(getLength([1, 2, 3, 4, 5])); // prints 5
function getLength(arr) {
return arr.length;
}
console.log(getLength([1, 2, 3, 4, 5])); // prints 5
✨ 5. Mapping an array of numbers to an array of their squares:
const square = n => n * n;
const squareAll = arr => arr.map(square);
console.log(squareAll([1, 2, 3, 4, 5])); // prints [1, 4, 9, 16, 25]
function square(n) {
return n * n;
}
function squareAll(arr) {
return arr.map(square);
}
console.log(squareAll([1, 2, 3, 4, 5])); // prints [1, 4, 9, 16, 25]